Home > Electronics > Bike Lamp > Programming
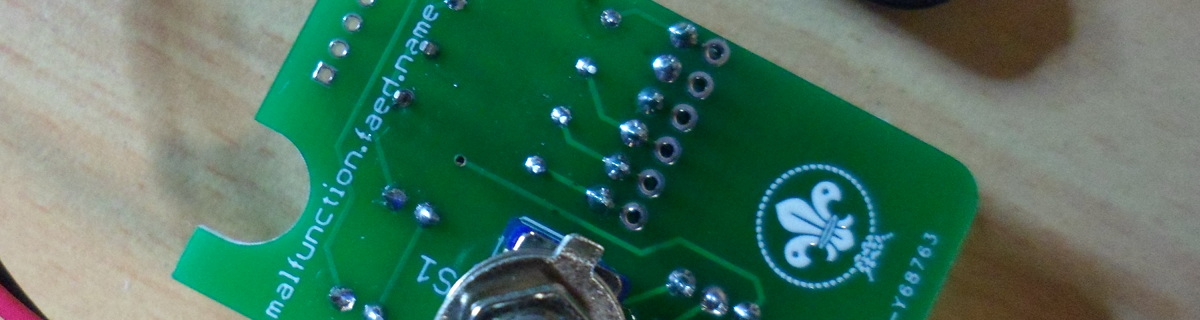
Arduino Coding for the Bike Lamp
These note refer to the source code listing below.
Coding Notes
In the Arduino programming environment, your program is called a sketch. There are two predifined functions used in every sketch: setup() and loop().
The setup() function is called when a sketch starts. Use it to initialize variables, pin modes, start using libraries, etc. The setup function will only run once, after each power up or reset of the board.
The loop() function does precisely what its name suggests, and loops continuously, allowing your program to change and respond as it runs. Code in the loop() section of your sketch is used to actively control the board.
The battery should not be connected when programming as power is provided through the USB plug.
To program the board, fire up the Arduino application. Compile and
upload the code using the
button.
Connect the board when instructed.
Commands Used
// Indicates a comment. Comments are not run as part of the program.
; Each line ends with a semicolon to signify the end of the command.
#define defines a macro. E.g. where LED1 appears in the code, it will be replaced with a 0.
pinMode(pin,mode); is used to set a pin as an input or an output
delay(x) pauses code execution for x milliseconds. (300 = .3 seconds)
digitalWrite(LED1, LOW); turns an output to an LED on or off. HIGH = on / LOW = off.
Programming Challenges
Speed up or slow down the Morse code. (Hint: dit_time controls the delay.)
Change the Morse code to spell your name. (Hint: International Morse code)
Blink all LEDs at once with a .5 second on / off time.
Blink each LED in sequence with an on time of .2 seconds.
Bike Lamp Code
// 2016 JOTA project for Sydney North Region Scouts. // Morse Code Timing // https://en.wikipedia.org/wiki/Morse_code // https://jota.uniq.com.au/radio/morsecode.html // Digispark ATTiny85 Boot Loader #define LED1 0 #define LED2 1 #define LED3 2 #define LED4 5 #define dit_time 200 #define dah_time dit_time * 3 void setup() { // put your setup code here, to run once: pinMode(LED1, OUTPUT); pinMode(LED2, OUTPUT); pinMode(LED3, OUTPUT); pinMode(LED4, OUTPUT); } // put your main code here, to run repeatedly: void loop() { // turn each LED on, in turn flash(LED1, 500); flash(LED2, 500); flash(LED3, 500); flash(LED4, 500); // make each LED flash a few times quickly flicker(LED1, 100, 4); flicker(LED2, 100, 4); flicker(LED3, 100, 4); flicker(LED4, 100, 4); delay(500); // Spell a short message in Morse Code // J dit(); dah(); dah(); dah(); letter_space(); // O dah(); dah(); dah(); letter_space(); // T dah(); letter_space(); // A dit(); dah(); letter_space(); word_space(); } // end loop() // Subroutines // Morse Dit void dit() { all_on(); delay(dit_time); all_off(); delay(dit_time); } // Morse Dah = 3 x Dit. void dah() { all_on(); delay(dah_time); all_off(); delay(dit_time); } // Letter Space = 3 x Dit void letter_space() { delay(dit_time * 3); } // Morse Word Space = 7 x Dit void word_space() { delay(dit_time * 7); } // All LEDs On. void all_on() { digitalWrite(LED1, HIGH); digitalWrite(LED2, HIGH); digitalWrite(LED3, HIGH); digitalWrite(LED4, HIGH); } // All LEDs Off. void all_off() { digitalWrite(LED1, LOW); digitalWrite(LED2, LOW); digitalWrite(LED3, LOW); digitalWrite(LED4, LOW); } // Flash LEDx on for a duration of wait ms. void flash(int pin, int wait) { digitalWrite(pin, HIGH); delay(wait); digitalWrite(pin, LOW); } // Flicker LEDx for period wait n times. void flicker(int pin, int wait, int times) { int i; for (i = 0; i <= times; i++) { flash(pin, wait); delay(wait); } }